GRAPHQL API Documentation
API (Application Programming Interface)
makes available resources of
You will find everything you need to integrate with your software.
(legacy) REST API documentation click here
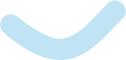
1. Resources and URLs
The new API
online-billing-service.com
is a GraphQL API, traverses and returns application data based on the schema definitions, independent of how the data is stored.
The schema defines an API's type system and all object relationships.
GraphQL official documentation.
For the examples in the Ruby language, we used the gem "graphql", "~> 1.10". With version 2 or newer, various errors may occur.
2. Sandbox system for API testing
To test the functions of the
online-billing-service.com
API we have developed a sandbox system.
It is available at:
https://sandbox.online-billing-service.com
The API key for testing is: 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829
The login data for the user corresponding to the above API key:
- Login address: https://sandbox.online-billing-service.com/users/sign_in
- Account name: sandbox
- Username: john.smith
- Password: j0HnsPsW!D
3. Authentication
API key is defined per user and it can be (re)generated from your account online-billing-service.com , under “My account” -> “API Key” Two authentication methods are available: HTTP Authentication (the API key will be used as a username, the password being ignored) Api_key parameter added to each request.
4. Results and errors
The result of a request signals via the returned HTTP status:
200 Success (after a GET, PUT, or DELETE successful request )
201 Created (after a POST successful request)
400 Resource Invalid (wrong formatted request)
401 Unauthorized
404 Resource Not Found
405 Method Not Allowed (The HTTP verb used is not supported for this resource)
422 Unprocessable Entity (The request was syntactically correct, but the requested changes are not valid)
500 Application Error (System error)
For syntactically correct requests, but that do not meet the validation criteria of the system, errors will be returned to the body of the response.
5. Fields and data types
The fields and data types can be seen in the Documentation Explorer on the right side of the GraphQL editor. Click here for an example.
6. Queries examples
6.1. Account (Model Account)
Account details
{
account {
id
name
companyAddress1
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of your account, please check the interactive documentation.
6.2. Client (Model client)
Client details
{
clients(id: "1064116516") {
id
uid
address
city
zip
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of clients, please check the interactive documentation.
Listing clients
{
clients(limit: 1, offset: 1) {
id
name
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of clients, please check the interactive documentation.
Searching clients
{
clients(id: "1064115995", name: "client name", email: "[email protected]") {
id
name
email
uid
address
city
zip
state
telephone
zip
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of searching clients, please check the interactive documentation.
Filtering clients
{
clients(where: "{\"_eq\": {\"name\": \"client name\", \"email\": \"[email protected]\"}}") {
id
name
email
uid
address
city
zip
state
telephone
zip
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of filtering clients, please check the interactive documentation.
Descending order clients
{
clients(orderBy: "{\"email\": \"desc\"}") {
id
name
email
uid
address
city
zip
state
telephone
zip
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of ordering clients, please check the interactive documentation.
Ascending order clients
{
clients(orderBy: "{\"email\": \"asc\"}") {
id
name
email
uid
address
city
zip
state
telephone
zip
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of ordering clients, please check the interactive documentation.
Add client
mutation {
createClient(
uid: "12345678",
name: "client name",
address: "client address",
city: "City name",
country: "DE"
) {
id
uid
name
address
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a client, please check the interactive documentation.
Modify client
mutation {
updateClient(
id: "1064116516",
name: "new name",
country: "DE",
uid: "3987985"
) {
id
uid
name
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a client, please check the interactive documentation.
Delete client
mutation {
deleteClient(id: "1064116516") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a client, please check the interactive documentation.
6.3. Invoice numbering schemes (Model Invoice Series)
Invoice numbering scheme details
{
invoiceSeries(id: "1061104946") {
id
createdAt
counterStart
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of invoice numbering schemes, please check the interactive documentation.
Listing invoice numbering scheme
{
invoiceSeries(limit: 1, offset: 1) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of invoice numbering schemes, please check the interactive documentation.
Searching invoice numbering scheme
{
invoiceSeries(year: "2021", counterStart: "1000") {
id
createdAt
counterStart
counterCurrent
prefix
year
sepparator
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of searching invoice numbering schemes, please check the interactive documentation.
Filtering invoice numbering scheme
{
invoiceSeries(where: "{\"_lte\": {\"year\": \"2036\"}}") {
id
createdAt
counterStart
counterCurrent
prefix
year
sepparator
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of filtering invoice numbering schemes, please check the interactive documentation.
Delete invoice numbering scheme
mutation {
deleteInvoiceSeries(id: "1061104946") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting an invoice numbering scheme, please check the interactive documentation.
Add invoice numbering scheme
mutation {
createInvoiceSeries(
counterStart: "1",
counterCurrent: "422",
year: "2025",
prefix: "qwerty"
) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding an invoice numbering scheme, please check the interactive documentation.
Modify invoice numbering scheme
mutation {
updateInvoiceSeries(
id: "1061104946",
prefix: "SER"
) {
id
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying an invoice numbering scheme, please check the interactive documentation.
6.4. Invoice (Model Invoice)
Invoice details
{
invoices(id: "1065256351") {
id
createdAt
type
lowerAnnotation
upperAnnotation
documentPositions {
id
}
receipts {
id
}
inputCurrency
payments {
id
}
actionEvents {
id
}
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of invoices, please check the interactive documentation.
Listing invoices
{
invoices(limit: 1, offset: 1) {
id
lowerAnnotation
upperAnnotation
documentState
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of invoices, please check the interactive documentation.
Searching invoices
{
invoices(documentSeriesId: "1061104955") {
id
lowerAnnotation
upperAnnotation
documentState
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of searching invoices, please check the interactive documentation.
Filtering invoices
{
invoices(where: "{\"_eq\": {\"cachedTotal\": \"145.2\", \"vatType\": \"1\"}}") {
id
lowerAnnotation
upperAnnotation
documentState
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of filtering invoices, please check the interactive documentation.
Descending order invoices
{
invoices(orderBy: "{\"createdAt\": \"desc\"}") {
id
lowerAnnotation
upperAnnotation
documentState
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of ordering invoices, please check the interactive documentation.
Ascending order invoices
{
invoices(orderBy: "{\"createdAt\": \"asc\"}") {
id
lowerAnnotation
upperAnnotation
documentState
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of ordering invoices, please check the interactive documentation.
Delete invoices
mutation {
deleteInvoice(id: "1065256351") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting an invoice, please check the interactive documentation.
Add invoices
mutation {
createInvoice(
currency: "EUR",
clientId: "1064116516",
documentSeriesId: "1061104955",
documentDate: "2026-04-04",
exchangeRate: "4.55",
lowerAnnotation: "lower annotation example",
upperAnnotation: "upper annotation example",
documentSeriesCounter: "475",
vatType: "1",
delegateId: "525664504",
displayTransportData: "1",
documentPositions: [{
description: "BASIC SUBSCRIPTION",
unit: "months",
unitCount: "12",
price: "12",
productCode: "66XXH663496H",
vat: "19"
}]) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding an invoice, please check the interactive documentation.
Modify invoices
mutation {
updateInvoice(
id: "1065256351",
exchangeRate: "4.5",
currency: "EUR",
documentPositions: [{
description: "product",
unit: "-",
unitCount: "1",
total: "0.0",
productCode: "none",
vat: "20.0",
position: "0"
}]) {
id
currency
exchangeRate
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying an invoice, please check the interactive documentation.
Send invoice through e-mail
mutation {
sendDocument(to: "random_email@random_service.domain", documentId: "1065256351", body: "your invoice", bcc: "random_email2@random_service.domain") {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects for sending invoices through e-mail, please check the interactive documentation.
Convert invoice in PDF format encoded as Base64 (it works for all types of documents)
{
invoices(id: "1065256351") {
id
pdfContent
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects convert invoices in PDF format, please check the interactive documentation.
Send invoice through e-mail
mutation {
sendDocument(to: "random_email@random_service.domain", documentId: "1065256351" body: "your invoice") {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects for invoices sending, please check the interactive documentation.
6.5. Notice numbering schemes (Model Notice Series)
Notice numbering scheme details
{
noticeSeries(id: "1061104953") {
id
createdAt
counterStart
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of notice numbering schemes, please check the interactive documentation.
Delete notice numbering scheme
mutation {
deleteNoticeSeries(id: "1061104953") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a notice numbering scheme, please check the interactive documentation.
Add notice numbering scheme
mutation {
createNoticeSeries(counterStart: "1",
counterCurrent: "422",
year: "2025",
prefix: "qwerty") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of creating a notice numbering scheme, please check the interactive documentation.
Modify notice numbering scheme
mutation {
updateNoticeSeries(id: "1061104953", prefix: "SER") {
id
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a notice numbering scheme, please check the interactive documentation.
6.6. Notices (Model Notice)
Notice details
{
notices(id: "1065256393") {
id
createdAt
type
documentPositions {
id
}
inputCurrency
actionEvents {
id
}
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of notices, please check the interactive documentation.
Delete notice
mutation {
deleteNotice(id: "1065256393") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a notice, please check the interactive documentation.
Add notice
mutation {
createNotice(
currency: "EUR",
clientId: "1064116516",
documentSeriesId: "1061104953",
documentDate: "2025-02-09",
exchangeRate: "4.55",
documentSeriesCounter: "423",
vatType: "1",
delegateId: "525664504",
displayTransportData: "1",
documentPositions: [{
description: "BASIC SUBSCRIPTION",
unit: "months",
unitCount: "12",
price: "12",
productCode: "66XXH663496H",
vat: "19"
}]) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of creating a notice, please check the interactive documentation.
Modify notice
mutation {
updateNotice(id: "1065256393",
exchangeRate: "4.5",
currency: "EUR") {
id
currency
exchangeRate
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a notice, please check the interactive documentation.
6.7. Payments (Model Payment)
Payment details
{
payments(id: "969663972") {
id
createdAt
description
currency
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of payments, please check the interactive documentation.
Add payment
mutation {
createPayment(description: "payment description",
currency: "EUR",
paymentDate: "2025-07-11",
proformaInvoiceId: "1065256380"
invoiceId: "1065256351",
amount: "200"
) {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a payment, please check the interactive documentation.
Modify payment
mutation {
updatePayment(id: "969663972",
description: "new description",
currency: "EUR"
) {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a payment, please check the interactive documentation.
Delete payment
mutation {
deletePayment(id: "969663972") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a payment, please check the interactive documentation.
6.8. Products (Model Product)
Product details
{
products(id: "741342701") {
id
createdAt
description
currency
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of products, please check the interactive documentation.
Add products
mutation {
createProduct(description: "product description",
currency: "EUR"
) {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a product, please check the interactive documentation.
Modify products
mutation {
updateProduct(id: "741342701",
description: "new description",
currency: "EUR") {
id
description
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a product, please check the interactive documentation.
Delete products
mutation {
deleteProduct(id: "741342701") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a product, please check the interactive documentation.
6.9. Proforma invoice numbering schemes (Model Proforma Invoice Series)
Proforma invoice numbering scheme details
{
proformaInvoiceSeries(id: "1061104957") {
id
createdAt
counterStart
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of proforma invoice numbering schemes, please check the interactive documentation.
Add proforma invoice numbering scheme
mutation {
createProformaInvoiceSeries(
counterStart: "1",
counterCurrent: "422",
year: "2025",
prefix: "qwerty"
) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a proforma invoice numbering scheme, please check the interactive documentation.
Modify proforma invoice numbering scheme
mutation {
updateProformaInvoiceSeries(id: "1061104957", prefix: "SER") {
id
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a proforma invoice numbering scheme, please check the interactive documentation.
Delete proforma invoice numbering scheme
mutation {
deleteProformaInvoiceSeries(id: "1061104957") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a proforma invoice numbering scheme, please check the interactive documentation.
6.10. Proforma invoice (Model Proforma Invoice)
Proforma invoice details
{
proformaInvoices(id: "1065256380") {
id
createdAt
type
documentPositions {
id
}
inputCurrency
payments {
id
}
actionEvents {
id
}
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of proforma invoices, please check the interactive documentation.
Delete proforma invoice
mutation {
deleteProformaInvoice(id: "1065256380") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a proforma invoice, please check the interactive documentation.
Add proforma invoice
mutation {
createProformaInvoice(
currency: "EUR",
clientId: "1064116516",
documentSeriesId: "1061104957",
documentDate: "2026-06-01",
exchangeRate: "4.55",
documentSeriesCounter: "423",
vatType: "1",
delegateId: "525664504",
displayTransportData: "1",
documentPositions: [{
description: "BASIC SUBSCRIPTION",
unit: "months",
unitCount: "12",
price: "12",
productCode: "66XXH663496H",
vat: "19"
}]) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a proforma invoice, please check the interactive documentation.
Modify proforma invoice
mutation {
updateProformaInvoice(id: "1065256380", exchangeRate: "4.5", currency: "EUR") {
id
currency
exchangeRate
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a proforma invoice, please check the interactive documentation.
6.11. Receipt numbering scheme (Receipt Numbering Scheme)
Receipt numbering scheme details
{
receiptSeries(id: "1061104941") {
id
createdAt
counterStart
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of receipt numbering schemes, please check the interactive documentation.
Delete receipt numbering scheme
mutation {
deleteReceiptSeries(id: "1061104941") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a receipt numbering scheme, please check the interactive documentation.
Add receipt numbering scheme
mutation {
createReceiptSeries(counterStart: "1",
counterCurrent: "422",
year: "2025",
prefix: "qwerty"
) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a receipt numbering scheme, please check the interactive documentation.
Modify receipt numbering scheme
mutation {
updateReceiptSeries(id: "1061104941", prefix: "SER") {
id
prefix
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a receipt numbering scheme, please check the interactive documentation.
6.12. Receipt (Model Receipt)
Receipt
{
receipts(id: "1065256370") {
id
createdAt
type
invoiceDate
userFirstName
actionEvents {
id
}
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of receipts, please check the interactive documentation.
Delete receipt
mutation {
deleteReceipt(id: "1065256370") {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of deleting a receipt, please check the interactive documentation.
Add receipt
mutation {
createReceipt(
currency: "EUR",
clientId: "1064116516",
documentSeriesId: "1061104941",
documentDate: "0000-04-07",
documentSeriesCounter: "21123333",
receiptAmount: "23",
invoiceId: "1065256351",
vatType: "1",
delegateId: "525664504",
displayTransportData: "false",
invoiceDate: "2025-04-25"
) {
id
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of adding a receipt, please check the interactive documentation.
Modify receipt
mutation {
updateReceipt(id: "1065256370",
exchangeRate: "4.5",
currency: "EUR"
) {
id
currency
exchangeRate
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of modifying a receipt, please check the interactive documentation.
6.13. Users (Model User)
Details about users account
{
users(id: "525664504") {
id
firstName
lastName
}
}
curl -i -H 'Content-Type: application/json' -u 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829:x -X POST -d '{"query": "{{code_example}}"}' https://sandbox.online-billing-service.com/graphql
<?php
require_once('graphql-settings.php');
define('BASE_URL', 'https://sandbox.online-billing-service.com/graphql');
define('API_KEY', '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829');
$ch = curl_init();
require_once('graphql-functions.php');
$url = BASE_URL;
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, API_KEY . ":x");
curl_setopt($ch, CURLOPT_URL, $url );
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json')
);
curl_setopt($ch, CURLOPT_POSTFIELDS, '{"query": "{{code_example}}"}');
$result = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
echo($httpCode . "\n" . $result . "\n");
curl_close($ch);
#!/usr/bin/env ruby
# graphql gem version needed: "graphql", "~> 1.10"
require "base64"
require 'graphlient'
base_url = 'https://sandbox.online-billing-service.com/graphql'
api_key = '91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829'
encoded_credentials = ::Base64.strict_encode64("#{api_key}:")
headers = { Authorization: "Basic #{encoded_credentials}" }
graphql_client = Graphlient::Client.new(base_url, { headers: headers })
query_string = <<~GRAPHQL
{{code_example}}
GRAPHQL
response = graphql_client.query query_string
puts response.data.to_h
using System;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Collections.Generic;
using System.Text;
namespace c_
{
class Program
{
static void Main(string[] args)
{
var query = "{{code_example}}";
var baseUri = "https://sandbox.online-billing-service.com/graphql";
var apiKey = "91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829";
var queryParams = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("query", query)
});
var client = new HttpClient();
var authHeader = Convert.ToBase64String(Encoding.UTF8.GetBytes(apiKey + ":"));
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", authHeader);
var response = client.PostAsync(baseUri, queryParams).Result;
var responseString = response.Content.ReadAsStringAsync();
if (response.IsSuccessStatusCode)
{
System.Console.WriteLine(response);
System.Console.WriteLine(responseString.ToString());
foreach (var character in responseString.Result)
System.Console.Write(character);
System.Console.WriteLine();
}
}
}
}
import java.net.*;
import java.io.*;
import java.util.*;
import java.nio.charset.StandardCharsets;
public class Graph {
public static void main(String[] args) throws MalformedURLException {
String query = "{\"query\":\"{{code_example}} \" }";
byte[] postData = query.getBytes(StandardCharsets.UTF_8);
String apiKey = "743b27b9e2ba33269bca4506f858637739fca1f60562132c5b5463e40e4a";
URL serverUrl = new URL("https://sandbox.online-billing-service.com/graphql");
String basicAuthPayload = "Basic " + Base64.getEncoder().encodeToString((apiKey + ":").getBytes());
BufferedReader httpResponseReader = null;
try {
HttpURLConnection urlConnection = (HttpURLConnection) serverUrl.openConnection();
urlConnection.setDoOutput(true);
urlConnection.setRequestMethod("POST");
urlConnection.setRequestProperty("Content-Type", "application/json");
urlConnection.addRequestProperty("Authorization", basicAuthPayload);
try (DataOutputStream wr = new DataOutputStream(urlConnection.getOutputStream())) {
wr.write(postData);
wr.flush();
wr.close();
}
httpResponseReader =
new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
String lineRead;
while((lineRead = httpResponseReader.readLine()) != null) {
System.out.println(lineRead);
}
} catch (IOException ioe) {
ioe.printStackTrace();
} finally {
if (httpResponseReader != null) {
try {
httpResponseReader.close();
} catch (IOException ioe) {
// Close quietly
}
}
}
}
}
For a detailed list of fields, relations and imbricated objects of users, please check the interactive documentation.
GRAPHQL API endpoint: https://sandbox.online-billing-service.com/graphql
API Key: 91b4e03e6d4a07c088921a07ab8e844c7508092cf1645b32657d6c4b5829